Spring Boot + Activiti Script Task Example
This page will walk through Spring Boot + Activiti Script Task example. A script task is an automatic activity. When process execution arrives at the script task, the corresponding script is executed. A script task is visualized as a typical BPMN 2.0 task (rounded rectangle
), with a small script icon in the top-left corner of the rectangle.
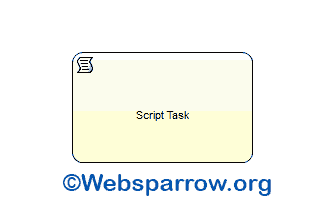
Activiti workflow engine supports two types of script:
- Groovy
- javascript
Note: Don’t confuse about JavaScript (we are not going to
alert/console
something on the web browser), it’s all about Java scripting, javascript engine that is embedded in the JDK, using the ScriptEngine class in the JDK. In JDK 6/7 this was Rhino, in JDK 8 this is Nashorn.
1. Technologies Used
Find the list of all technologies used in this application.
- STS 4
- JDK 8
- Spring Boot 1.5.20.RELEASE
- Activiti 5.22.0
- H2 Database
- groovy-all-2.4.16.jar
Similar Posts:
2. Dependencies Required
To make it work, make sure these following dependencies are available in your build path. Add the following to your pom.xml.
<properties>
<java.version>1.8</java.version>
<activiti.version>5.22.0</activiti.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.activiti</groupId>
<artifactId>activiti-spring-boot-starter-basic</artifactId>
<version>${activiti.version}</version>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
<dependency>
<groupId>org.codehaus.groovy</groupId>
<artifactId>groovy-all</artifactId>
</dependency>
</dependencies>
3. Groovy Script Task
To execute the Groovy script in the workflow, a script task is defined by specifying the attribute scriptFormat="groovy"
and the statement must be in the script
tag.
<scriptTask id="groovyScriptTask" name="Groovy Script Task" scriptFormat="groovy">
<script>
println '**** Groovy Script Task executed successfully ****'
</script>
</scriptTask>
3.1 BPMN Process Definition
Drop the BPMN 2.0 process definition into the src/main/resources/processes folder. See the complete BPMN process definition for Groovy script task.
<?xml version="1.0" encoding="UTF-8"?>
<definitions xmlns="http://www.omg.org/spec/BPMN/20100524/MODEL" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:activiti="http://activiti.org/bpmn"
xmlns:bpmndi="http://www.omg.org/spec/BPMN/20100524/DI" xmlns:omgdc="http://www.omg.org/spec/DD/20100524/DC"
xmlns:omgdi="http://www.omg.org/spec/DD/20100524/DI" typeLanguage="http://www.w3.org/2001/XMLSchema"
expressionLanguage="http://www.w3.org/1999/XPath" targetNamespace="http://www.activiti.org/test">
<process id="groovy-script-task-process" name="Activiti Script Task using Groovy" isExecutable="true">
<startEvent id="startevent1" name="Start"></startEvent>
<scriptTask id="groovyScriptTask" name="Groovy Script Task" scriptFormat="groovy" activiti:autoStoreVariables="false">
<script>
println '**** Groovy Script Task executed successfully ****'
</script>
</scriptTask>
<sequenceFlow id="flow1" sourceRef="startevent1" targetRef="groovyScriptTask"></sequenceFlow>
<endEvent id="endevent1" name="End"></endEvent>
<sequenceFlow id="flow2" sourceRef="groovyScriptTask" targetRef="endevent1"></sequenceFlow>
</process>
</definitions>
The above BPMN process definition gives you the below-visualized diagram:
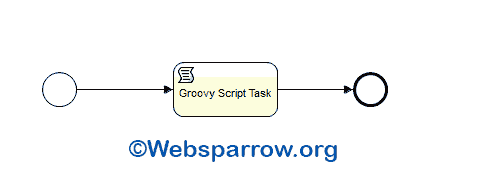
3.2 Execute it
Create a class which autowired the RuntimeService
and execute the process by its process instance key.
package org.websparrow.activiti.controller;
import org.activiti.engine.RuntimeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/activiti")
public class ScriptTaskController {
@Autowired
private RuntimeService runtimeService;
@GetMapping("script-task/groovy")
public String groovyScritTask() {
runtimeService.startProcessInstanceByKey("groovy-script-task-process");
return "check the console log...";
}
}
Output: Hit the http://localhost:8080/activiti/script-task/groovy URL in your web browser and check your IDE console log. You will get the:
**** Groovy Script Task executed successfully ****
4. javascript Script Task
A script task is defined by specifying the attribute scriptFormat="javascript"
and the statement must be in the script
tag.
<scriptTask id="jsScriptTask" name="JS Script Task" scriptFormat="javascript" >
<script>
java.lang.System.out.println("Hello Websparrow.org");
</script>
</scriptTask>
OR
<scriptTask id="jsScriptTask" name="JS Script Task" scriptFormat="javascript" >
<script>
var my = "Activiti is an open-source workflow engine.";
print(my);
</script>
</scriptTask>
4.1 BPMN Process Definition
See the complete BPMN process definition for javascript script task.
<?xml version="1.0" encoding="UTF-8"?>
<definitions xmlns="http://www.omg.org/spec/BPMN/20100524/MODEL" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:activiti="http://activiti.org/bpmn"
xmlns:bpmndi="http://www.omg.org/spec/BPMN/20100524/DI" xmlns:omgdc="http://www.omg.org/spec/DD/20100524/DC"
xmlns:omgdi="http://www.omg.org/spec/DD/20100524/DI" typeLanguage="http://www.w3.org/2001/XMLSchema"
expressionLanguage="http://www.w3.org/1999/XPath" targetNamespace="http://www.activiti.org/test">
<process id="js-script-task-process" name="Activiti Script Task using Groovy" isExecutable="true">
<startEvent id="startevent1" name="Start"></startEvent>
<scriptTask id="jsScriptTask" name="JS Script Task" scriptFormat="javascript" activiti:autoStoreVariables="false">
<script>
<!-- java.lang.System.out.println("Hello Websparrow.org"); -->
var my = "Activiti is an open-source workflow engine.";
print(my);
</script>
</scriptTask>
<sequenceFlow id="flow1" sourceRef="startevent1" targetRef="jsScriptTask"></sequenceFlow>
<endEvent id="endevent1" name="End"></endEvent>
<sequenceFlow id="flow2" sourceRef="jsScriptTask" targetRef="endevent1"></sequenceFlow>
</process>
</definitions>
The above BPMN process definition gives you the below-visualized diagram:
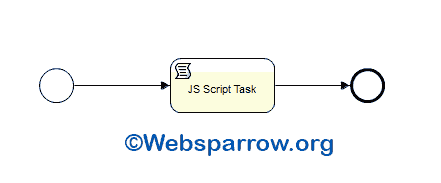
4.2 Execute it
package org.websparrow.activiti.controller;
import org.activiti.engine.RuntimeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/activiti")
public class ScriptTaskController {
@Autowired
private RuntimeService runtimeService;
@GetMapping("script-task/js")
public String jsScritTask() {
runtimeService.startProcessInstanceByKey("js-script-task-process");
return "check the console log...";
}
}
Output: Hit the http://localhost:8080/activiti/script-task/js URL in your web browser and check your IDE console log. You will get the:
Activiti is an open-source workflow engine.
Download Source Code: spring-boot-activiti-script-task-example.zip