File Handling Example in Java
This tutorial will explain “How to Handle File
in Java”. There are many ways to handle or manipulate the file in Java. Most of the I/O Streams classes are in the java.io
package.
An I/O Stream represents an input source or an output destination. A stream can represent many different kinds of sources and destinations, including disk files, devices, other programs, and memory arrays.
A program uses an input stream to read data from a source, one item at a time :
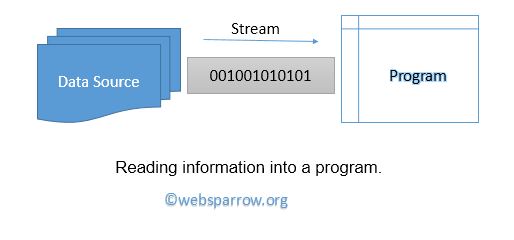
A program uses an output stream to write data to a destination, one item at a time :
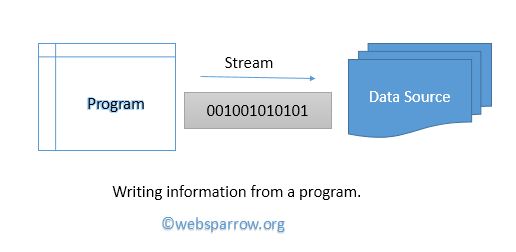
Byte Streams
Byte streams classes perform 8-bit bytes of input and output. All byte stream classes are descended from InputStream and OutputStream.
Read File using Byte Streams
This example will explain how to read a File
and display the file on the output console. This example uses the FileInputStream
class to read the file.
FileInputStreamExample.java
package org.websparrow.file;
import java.io.FileInputStream;
public class FileInputStreamExample {
public static void main(String[] args) {
try {
int x;
FileInputStream fileInputStream = new FileInputStream("F:/WebSparrow.txt");
while ((x = fileInputStream.read()) != -1) {
System.out.print((char) x);
}
fileInputStream.close();
System.out.println("\n\nTask Completed");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Read and Write File using Byte Streams
This example will explain how to read a File
and write it into another File
. We are uses the FileInputStream
class to read the file and FileOutputStream
to write the file.
FileOutputStreamExample.java
package org.websparrow.file;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class FileOutputStreamExample {
public static void main(String[] args) {
try {
int x;
FileInputStream fileInputStream = new FileInputStream("F:/WebSparrow.txt");
FileOutputStream fileOutputStream = new FileOutputStream("F:/ws.txt");
while ((x = fileInputStream.read()) != -1) {
fileOutputStream.write(x);
}
fileOutputStream.close();
fileInputStream.close();
System.out.println("\n\nTask Completed");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Character Streams
Character stream I/O automatically translates this internal format to and from the local character set. All character stream classes are descended from Reader and Writer.
Read File using Character Streams
This example will explain how to read a File
and display the file on the output console. This example uses the FileReader
class to read the file.
ReadFile.java
package org.websparrow.file;
import java.io.FileReader;
public class ReadFile {
public static void main(String[] args) {
try {
int line;
FileReader fileReader = new FileReader("F:/WebSparrow.txt");
while ((line = fileReader.read()) != -1) {
System.out.print((char) line);
}
fileReader.close();
System.out.println("\nTask Completed");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Write File using Character Streams
This example will explain how to write a File
. This example uses the FileWriter
class to write the file.
WriteFile.java
package org.websparrow.file;
import java.io.FileWriter;
public class WriteFile {
public static void main(String[] args) {
try {
FileWriter fileWriter = new FileWriter("F:/copyright.txt");
fileWriter.write("Welcome to WebSparrow.org !");
fileWriter.close();
System.out.println("done");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Read and Write File using Character Streams
This example will explain how to read a File
and write it into another File
. We are uses the FileReader
class to read the file and FileWriter
to write the file.
ReadWriteFile.java
package org.websparrow.file;
import java.io.FileReader;
import java.io.FileWriter;
public class ReadWriteFile {
public static void main(String[] args) {
try {
int line;
FileReader fileReader = new FileReader("F:/WebSparrow.txt");
FileWriter fileWriter = new FileWriter("F:/Hello.txt");
while ((line = fileReader.read()) != -1) {
fileWriter.write(line);
}
fileReader.close();
fileWriter.close();
System.out.println("Task Completed");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Buffered Streams
Buffered input streams read data from a memory area known as a buffer; the native input API is called only when the buffer is empty. Similarly, buffered output streams write data to a buffer, and the native output API is called only when the buffer is full.
BuffredInputOutputStream.java
package org.websparrow.file;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class BuffredInputOutputStream {
public static void main(String[] args) {
try {
int x;
BufferedInputStream bufferedInputStream = new BufferedInputStream(new FileInputStream("BisBos.java"));
BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(new FileOutputStream("ws.txt"));
while ((x = bufferedInputStream.read()) != -1) {
System.out.print((char) x);
bufferedOutputStream.write(x);
}
bufferedOutputStream.close();
bufferedInputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
How to Delete a File
To delete any file from your machine using Java program use File.delete()
. If file is deleted then it will return true otherwise false. The delete()
will throw NoSuchFileException
if file not found. For more detail see the example.
DeleteFile.java
package org.websparrow.file;
import java.io.File;
public class DeleteFile {
public static void main(String[] args) {
try {
File file = new File("F:/Hello.txt");
if (file.delete()) {
System.out.println("SUCCESS");
} else {
System.out.println("FAILURE");
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
How to make File write Protected
To make any file write protected in Java use the setReadOnly()
method of File class.
WriteProtectedFile.java
package org.websparrow.file;
import java.io.File;
public class WriteProtectedFile {
public static void main(String[] args) {
try {
File file = new File("F:/copyright.txt");
if (file.setReadOnly()) {
System.out.println("Success");
} else {
System.out.println("Failure");
}
} catch (Exception e) {
e.printStackTrace();
}
}
}