Show and Hide Password using jQuery and JavaScript
This page will walk through how to toggle or show and hide password using jQuery and JavaScript in HTML form. Show and hide password enhanced the user experience while Sign In or Sign Up on the web page.
It can be achieved by using jQuery or vanilla JavaScript. So Let’s start…
Similar Posts:
Library Used
Find the list of all libraries used in this demo.
- Bootstrap 4
- Font Awesome Icon
- jQuery
- and JavaScript
Design a login form
Design the login form using Bootstrap 4 CSS and HTML 5. Add eye icon from Font Awesome library and custom CSS if needed.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>Login - Websparrow.org</title>
<!-- Bootstrap core CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<!-- Custom styles for this page -->
<link href="style.css" rel="stylesheet">
<!-- Fontawesome icon library -->
<script src="https://kit.fontawesome.com/5d0d55aa82.js"></script>
</head>
<body>
<form class="form-signin">
<div class="text-center mb-4">
<img class="mb-4" src="https://websparrow.org/wp-content/themes/websparrow/images/branding/ws-1.png" alt="Websparrow.org">
</div>
<div class="form-label-group">
<input type="text" id="userId" class="form-control" placeholder="User id" required autofocus>
</div>
<div class="form-label-group input-group">
<input type="password" id="password" class="form-control" placeholder="Password" required>
<div class="input-group-append">
<span class="input-group-text">
<i id="eye" class="far fa-eye-slash"></i>
</span>
</div>
</div>
<button class="btn btn-lg btn-primary btn-block" type="button">Sign in</button>
</form>
</body>
</html>
Custom CSS used for this web page.
body { height: 100%;display: -ms-flexbox;display: flex;-ms-flex-align: center;
align-items: center;padding-top: 40px;padding-bottom: 40px;background-color: #f5f5f5;
}
.form-signin {
width: 100%;
max-width: 420px;
padding: 15px;
margin: auto;
}
.form-label-group {
position: relative;
margin-bottom: 1rem;
}
.form-label-group > input{
height: 3.125rem;
padding: .75rem;
}
i:hover {
cursor: pointer;
}
Now let’s jump to the actual piece of coding.
Show & Hide using JavaScript
Vanilla JavaScript is capable to toggle show and hide the password. In this case, we don’t require any external library but the problem with vanilla JavaScript is we have to write at least 10 lines of code which we don’t require.
To to that, create a function and get the attribute type of password field if type === "password"
change it to “text” and call this function onClick
event of eye button.
function showHidePwd() {
var input = document.getElementById("password");
if (input.type === "password") {
input.type = "text";
document.getElementById("eye").className = "far fa-eye";
} else {
input.type = "password";
document.getElementById("eye").className = "far fa-eye-slash";
}
}
Modified password field of the login form.
<div class="form-label-group input-group">
<input type="password" id="password" class="form-control" placeholder="Password" required>
<div class="input-group-append">
<span class="input-group-text">
<i id="eye" class="far fa-eye-slash" onclick="showHidePwd();"></i>
</span>
</div>
</div>
Show & Hide using jQuery
jQuery is an open-source JavaScript library designed to simplify HTML DOM tree traversal and manipulation. It is widely used and famous among the front-end developers. It reduced the boilerplates code that we have done using vanilla JavaScript. To start with jQuery, we need to add the jQuery library first.
Add the following inside the head
tag of the web page.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>Login - Websparrow.org</title>
<!-- Other CSS libraries -->
<!-- jQuery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<!-- Other JS libraries -->
</head>
And toggle show and hide the password, add the below code:
$(document).ready(function () {
$('#eye').click(function () {
$('#password').attr('type', $('#password').is(':password') ? 'text' : 'password');
if ($('#password').attr('type') === 'password') {
$('#eye').removeClass('fa-eye').addClass('fa-eye-slash');
} else {
$('#eye').removeClass('fa-eye-slash').addClass('fa-eye');
}
});
});
Finally, everything is set, open the login form, type some user id and password and click on eye button. Both JavaScript and jQuery gives the same result.
——————— hide password ———————
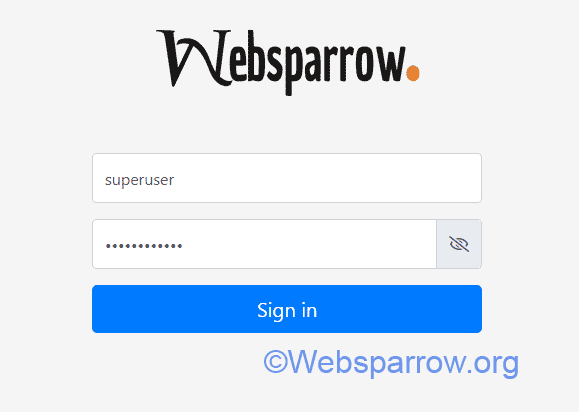
——————— show password ———————
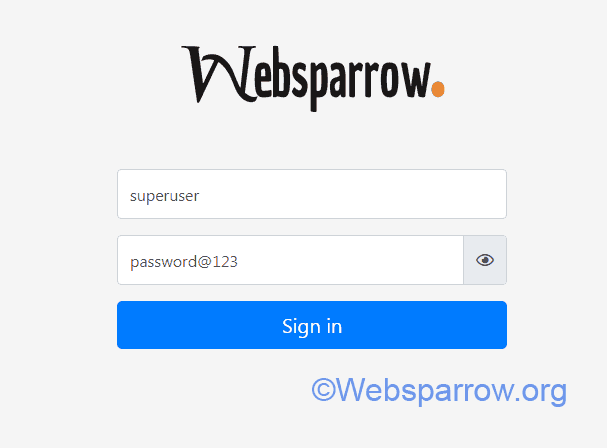
References
- Bootstrap CSS
- Font Awesome Icon
- jQuery Library
- jQuery show hide toggle example
- How to add and remove attribute in jQuery